【Git】GitHub ActionsでECSにCI/CDする方法
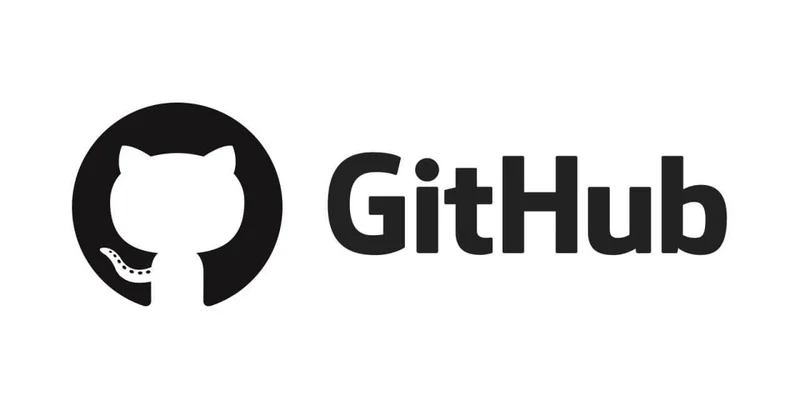
今回はGitHub ActionsでECSにCI/CDする方法の紹介です。
GitHub ActionsのCI/CD方法
「Actions」を押下して、ECSの「Configure」を押下します。
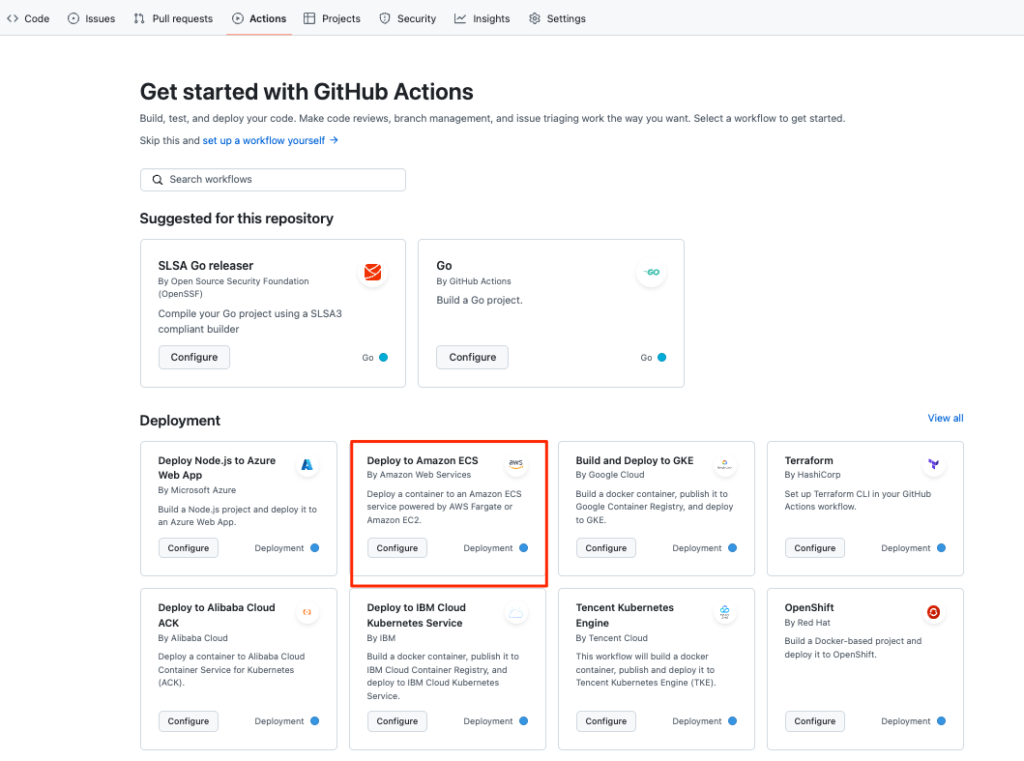
「Commit changes…」を押下してymlファイルをコミットします。
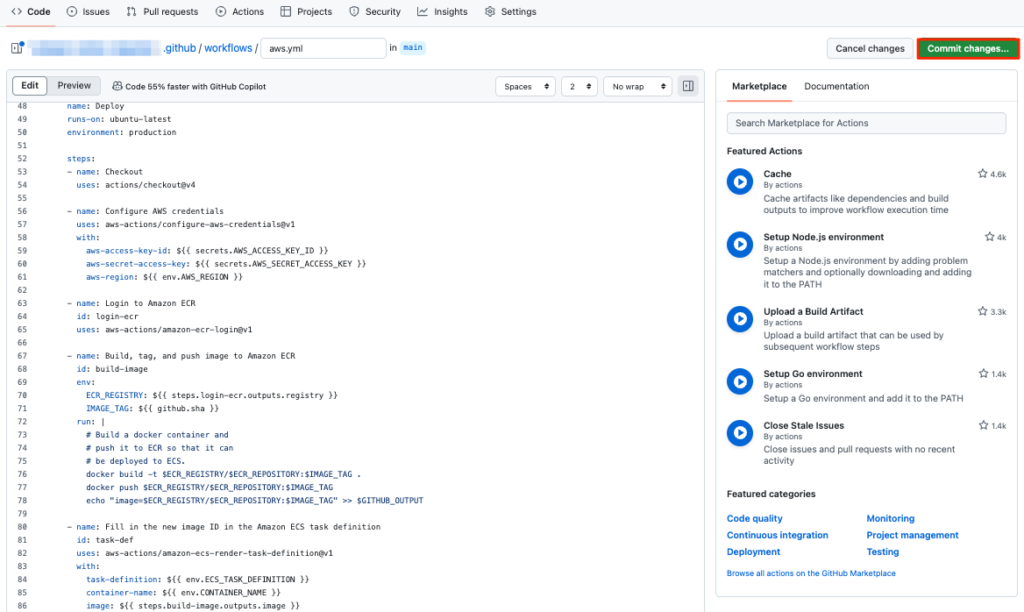
もし、mainとdevelopでどちらも環境を用意したい場合は、ymlファイルの名前をmainとdevelopに二つ作りましょう。
- main(master):本番環境
- develop:開発環境
main専用の全体のコード
# This workflow will build and push a new container image to Amazon ECR,
# and then will deploy a new task definition to Amazon ECS, when there is a push to the "main" branch.
#
# To use this workflow, you will need to complete the following set-up steps:
#
# 1. Create an ECR repository to store your images.
# For example: `aws ecr create-repository --repository-name my-ecr-repo --region us-east-2`.
# Replace the value of the `ECR_REPOSITORY` environment variable in the workflow below with your repository's name.
# Replace the value of the `AWS_REGION` environment variable in the workflow below with your repository's region.
#
# 2. Create an ECS task definition, an ECS cluster, and an ECS service.
# For example, follow the Getting Started guide on the ECS console:
# https://us-east-2.console.aws.amazon.com/ecs/home?region=us-east-2#/firstRun
# Replace the value of the `ECS_SERVICE` environment variable in the workflow below with the name you set for the Amazon ECS service.
# Replace the value of the `ECS_CLUSTER` environment variable in the workflow below with the name you set for the cluster.
#
# 3. Store your ECS task definition as a JSON file in your repository.
# The format should follow the output of `aws ecs register-task-definition --generate-cli-skeleton`.
# Replace the value of the `ECS_TASK_DEFINITION` environment variable in the workflow below with the path to the JSON file.
# Replace the value of the `CONTAINER_NAME` environment variable in the workflow below with the name of the container
# in the `containerDefinitions` section of the task definition.
#
# 4. Store an IAM user access key in GitHub Actions secrets named `AWS_ACCESS_KEY_ID` and `AWS_SECRET_ACCESS_KEY`.
# See the documentation for each action used below for the recommended IAM policies for this IAM user,
# and best practices on handling the access key credentials.
name: Deploy to Amazon ECS
on:
push:
branches: [ "main" ]
env:
AWS_REGION: MY_AWS_REGION # set this to your preferred AWS region, e.g. us-west-1
ECR_REPOSITORY: MY_ECR_REPOSITORY # set this to your Amazon ECR repository name
ECS_SERVICE: MY_ECS_SERVICE # set this to your Amazon ECS service name
ECS_CLUSTER: MY_ECS_CLUSTER # set this to your Amazon ECS cluster name
ECS_TASK_DEFINITION: MY_ECS_TASK_DEFINITION # set this to the path to your Amazon ECS task definition
# file, e.g. .aws/task-definition.json
CONTAINER_NAME: MY_CONTAINER_NAME # set this to the name of the container in the
# containerDefinitions section of your task definition
permissions:
contents: read
jobs:
deploy:
name: Deploy
runs-on: ubuntu-latest
environment: production
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Configure AWS credentials
uses: aws-actions/configure-aws-credentials@v1
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY_ID }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws-region: ${{ env.AWS_REGION }}
- name: Login to Amazon ECR
id: login-ecr
uses: aws-actions/amazon-ecr-login@v1
- name: Build, tag, and push image to Amazon ECR
id: build-image
env:
ECR_REGISTRY: ${{ steps.login-ecr.outputs.registry }}
IMAGE_TAG: ${{ github.sha }}
run: |
# Build a docker container and
# push it to ECR so that it can
# be deployed to ECS.
docker build -t $ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG .
docker push $ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG
echo "image=$ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG" >> $GITHUB_OUTPUT
- name: Fill in the new image ID in the Amazon ECS task definition
id: task-def
uses: aws-actions/amazon-ecs-render-task-definition@v1
with:
task-definition: ${{ env.ECS_TASK_DEFINITION }}
container-name: ${{ env.CONTAINER_NAME }}
image: ${{ steps.build-image.outputs.image }}
- name: Deploy Amazon ECS task definition
uses: aws-actions/amazon-ecs-deploy-task-definition@v1
with:
task-definition: ${{ steps.task-def.outputs.task-definition }}
service: ${{ env.ECS_SERVICE }}
cluster: ${{ env.ECS_CLUSTER }}
wait-for-service-stability: true
トリガー
mainブランチへのプッシュイベントがトリガーとなります。
on:
push:
branches: [ "main" ]
環境変数の設定
環境変数には、AWSリソース名や設定ファイルのパスが含まれます。
- AWS_REGION: AWSリージョン。
- ECR_REPOSITORY: Amazon ECRのリポジトリ名。
- ECS_SERVICE: Amazon ECSサービス名。
- ECS_CLUSTER: ECSクラスター名。
- ECS_TASK_DEFINITION: タスク定義ファイルのパス。
- CONTAINER_NAME: タスク定義のcontainerDefinitionsセクションにあるコンテナ名。
env:
AWS_REGION: MY_AWS_REGION
ECR_REPOSITORY: MY_ECR_REPOSITORY
ECS_SERVICE: MY_ECS_SERVICE
ECS_CLUSTER: MY_ECS_CLUSTER
ECS_TASK_DEFINITION: MY_ECS_TASK_DEFINITION
CONTAINER_NAME: MY_CONTAINER_NAME
AWS_REGION
リージョンは画面右上に表示されています。
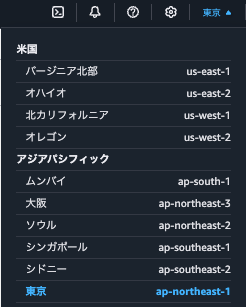
ECR_REPOSITORY
「ECS」のサイドバーから「ECR」のリポジトリを押下します。
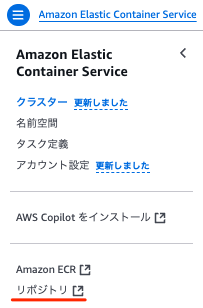
「ECR」画面に遷移するので、「Repositories」を押下してください。
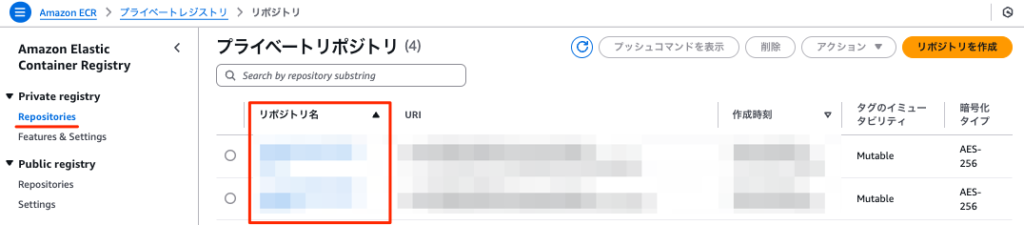
まだ作成してない場合はECRからリポジトリ作成してください。
リポジトリ名はGitのリポジトリ名ではなくECRのリポジトリURLは独自に設定できます。
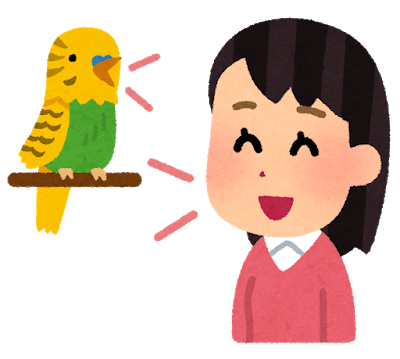
ECR(Elastic Container Registry)のリポジトリURLは、コンテナイメージを保存するためのURL であり、Docker CLIを使用してコンテナイメージをプッシュまたはプルする際に利用します。
<AWSアカウントID>.dkr.ecr.<リージョン>.amazonaws.com/<リポジトリ名>
dkrはDockerです。
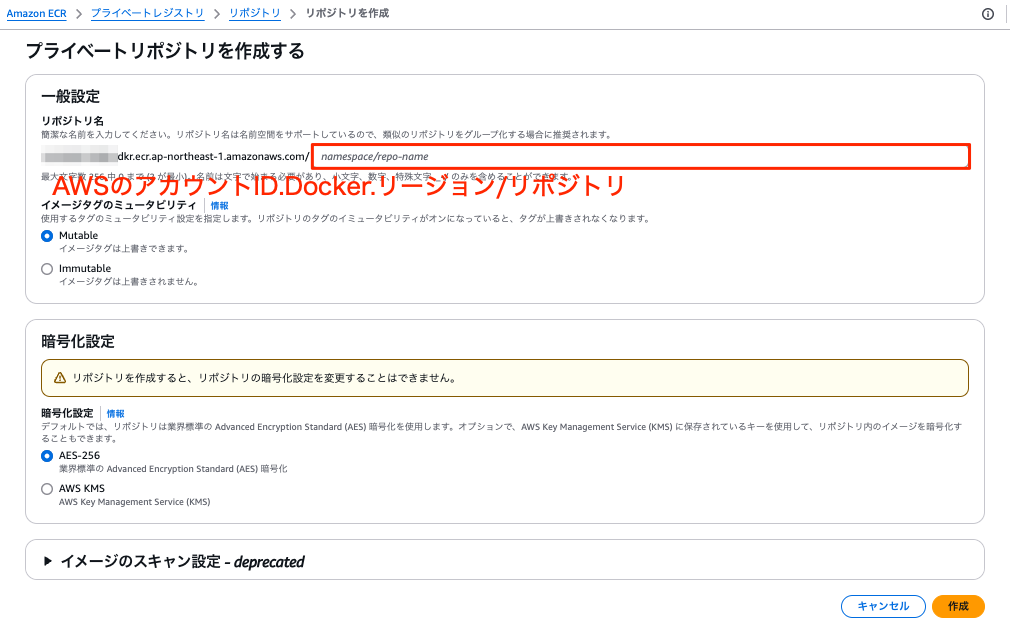
ECS_SERVICE
「ECS_SERVICE」は「Service name」に表示されています。
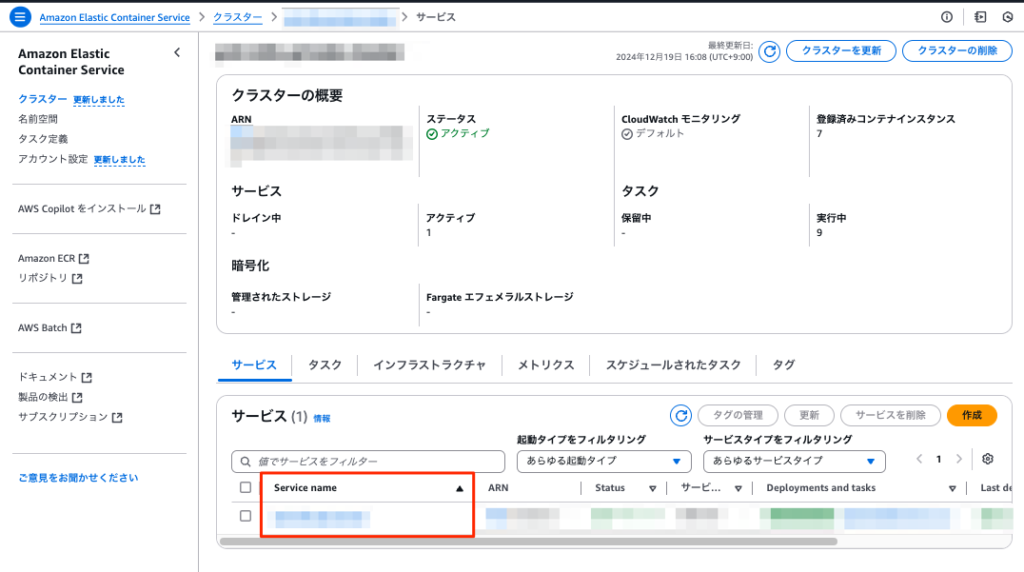
ECS_CLUSTERECSのクラスターはECSのクラスター作成時につけます。
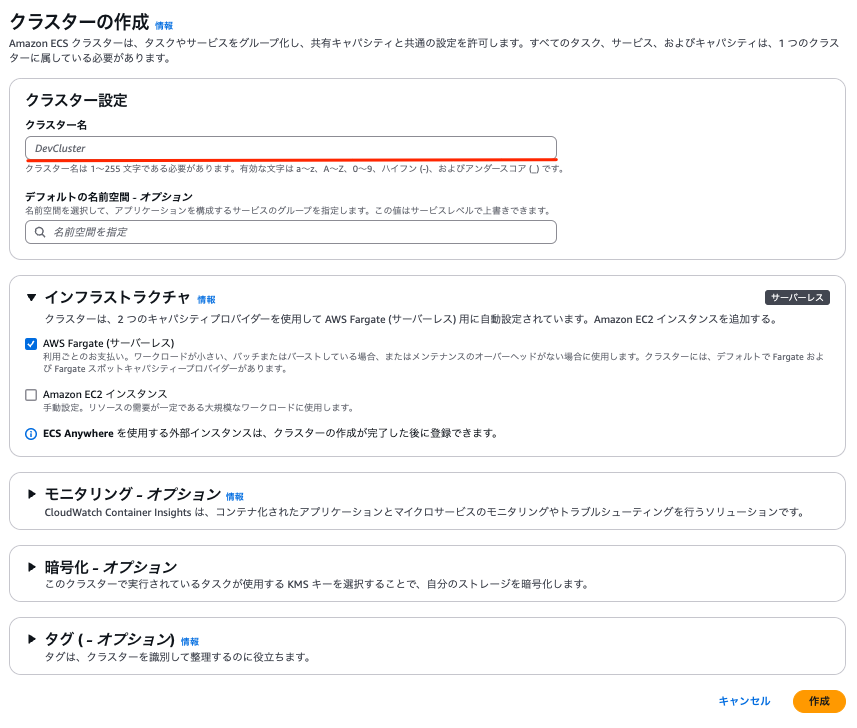
作成後に確認する場合は、ECSの画面開いたら表示されています。
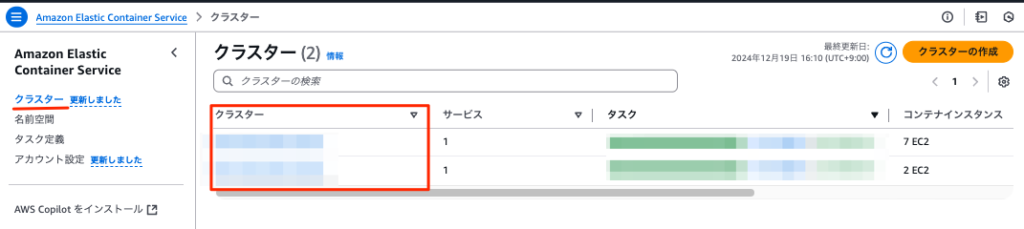
ECS_TASK_DEFINITION
タスク定義は「クラスター」の「Task definition」に表示されています。
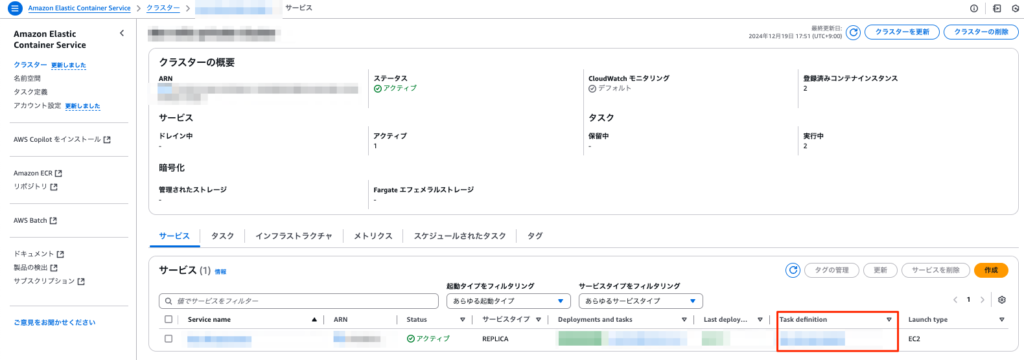
CONTAINER_NAME
AWS認証情報の設定
- AWSにアクセスするために必要な認証情報を設定します。
- AWS_ACCESS_KEY_ID と AWS_SECRET_ACCESS_KEY はGitHub Secretsから取得。
- name: Configure AWS credentials
uses: aws-actions/configure-aws-credentials@v1
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY_ID }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws-region: ${{ env.AWS_REGION }}
Amazon ECRへのログイン
- ECR(Elastic Container Registry)にログインします。
- ログイン情報をsteps.login-ecr.outputs.registryに保存。
- name: Login to Amazon ECR
id: login-ecr
uses: aws-actions/amazon-ecr-login@v1
Dockerイメージのビルドとプッシュ
- Dockerイメージのビルド:
- リポジトリとプッシュされたコミット(github.sha)をもとにタグを付けてイメージをビルド。
- ECRにプッシュ:
- ビルドしたイメージをECRにプッシュ。
- イメージパスの出力:
- プッシュしたイメージのパスをbuild-image.outputs.imageに保存。
- name: Build, tag, and push image to Amazon ECR
id: build-image
env:
ECR_REGISTRY: ${{ steps.login-ecr.outputs.registry }}
IMAGE_TAG: ${{ github.sha }}
run: |
docker build -t $ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG .
docker push $ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG
echo "image=$ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG" >> $GITHUB_OUTPUT
ECSタスク定義の更新
- タスク定義ファイル(ECS_TASK_DEFINITION)を元に、新しいDockerイメージを適用。
- コンテナ名(CONTAINER_NAME)とイメージパスを設定。
- name: Fill in the new image ID in the Amazon ECS task definition
id: task-def
uses: aws-actions/amazon-ecs-render-task-definition@v1
with:
task-definition: ${{ env.ECS_TASK_DEFINITION }}
container-name: ${{ env.CONTAINER_NAME }}
image: ${{ steps.build-image.outputs.image }}
ECSサービスへのデプロイ
- ECSサービスの更新:
- 更新されたタスク定義を使用して、指定されたECSサービス(ECS_SERVICE)をデプロイ。
- クラスター(ECS_CLUSTER)内で動作。
- 安定性の確認:
- サービスが安定するまで待機。
- name: Deploy Amazon ECS task definition
uses: aws-actions/amazon-ecs-deploy-task-definition@v1
with:
task-definition: ${{ steps.task-def.outputs.task-definition }}
service: ${{ env.ECS_SERVICE }}
cluster: ${{ env.ECS_CLUSTER }}
wait-for-service-stability: true
スポンサードサーチ
dev環境にCI/CDするには?
dev環境全体のyml
ブランチをdevelopに変更して、各変更箇所をdev用に変更します。
name: Deploy to Amazon ECS (dev)
on:
push:
branches: [ "develop" ] # dev環境用のブランチ
env:
AWS_REGION: MY_AWS_REGION # set this to your preferred AWS region, e.g. us-west-1
ECR_REPOSITORY: MY_ECR_REPOSITORY # set this to your Amazon ECR repository name
ECS_SERVICE: MY_ECS_SERVICE # set this to your Amazon ECS service name
ECS_CLUSTER: MY_ECS_CLUSTER # set this to your Amazon ECS cluster name
ECS_TASK_DEFINITION: MY_ECS_TASK_DEFINITION # set this to the path to your Amazon ECS task definition
# file, e.g. .aws/task-definition.json
CONTAINER_NAME: MY_CONTAINER_NAME # set this to the name of the container in the
# containerDefinitions section of your task definition
permissions:
contents: read
jobs:
deploy:
name: Deploy to ECS (dev)
runs-on: ubuntu-latest
environment: development
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Configure AWS credentials
uses: aws-actions/configure-aws-credentials@v1
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY_ID }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws-region: ${{ env.AWS_REGION }}
- name: Login to Amazon ECR
id: login-ecr
uses: aws-actions/amazon-ecr-login@v1
- name: Build, tag, and push image to Amazon ECR
id: build-image
env:
ECR_REGISTRY: ${{ steps.login-ecr.outputs.registry }}
IMAGE_TAG: ${{ github.sha }}
run: |
# DockerイメージをECRにプッシュ
docker build -t $ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG .
docker push $ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG
echo "image=$ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG" >> $GITHUB_OUTPUT
- name: Fill in the new image ID in the Amazon ECS task definition
id: task-def
uses: aws-actions/amazon-ecs-render-task-definition@v1
with:
task-definition: ${{ env.ECS_TASK_DEFINITION }}
container-name: ${{ env.CONTAINER_NAME }}
image: ${{ steps.build-image.outputs.image }}
- name: Deploy Amazon ECS task definition
uses: aws-actions/amazon-ecs-deploy-task-definition@v1
with:
task-definition: ${{ steps.task-def.outputs.task-definition }}
service: ${{ env.ECS_SERVICE }}
cluster: ${{ env.ECS_CLUSTER }}
wait-for-service-stability: true
dev環境にCI/CDするにあたり注意点
on
ブランチを developに設定
jobs.deploy.name
ジョブ名を Deploy to ECS (dev) に変更
steps
- AWS認証情報 (aws-access-key-id や aws-secret-access-key): 環境に合わせた dev 用の認証情報(GitHub Secrets)を利用します。
- タスク定義とコンテナの指定 (task-definition と container-name): env に指定された変数をそのまま使用しています。
task-definition: ${{ env.ECS_TASK_DEFINITION }}
container-name: ${{ env.CONTAINER_NAME }}
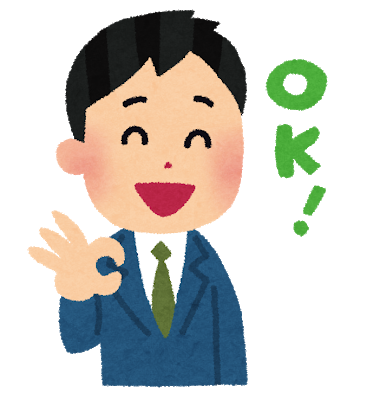
以上です。