【Laravel入門】interfaceとimplementsについて理解と実装を行う
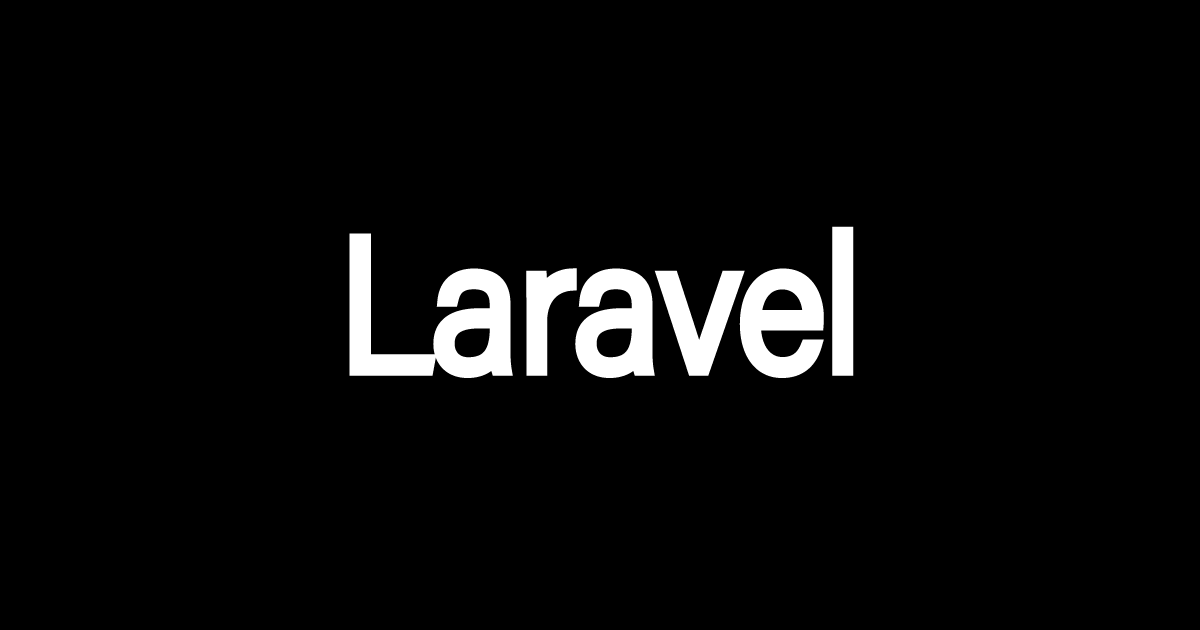
今回はinterfaceとimplementsについて理解と実装の紹介です。
Interfaceとは?
Interface(Interface)とは、クラスが実装すべきメソッドの宣言だけを含む型です。
具体的な実装は含まれておらず、メソッドのシグネチャ(名前、引数、返り値の型)だけを定義します。Interfaceを使うことで、異なるクラスが同じメソッドを持つことを保証できます。
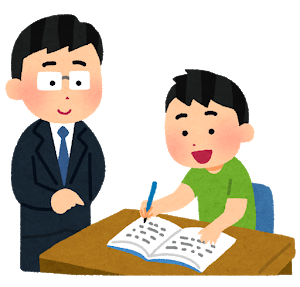
InterfaceとImplementsはセットで使います。
<?php
interface インターフェース名
{
public function 関数名(): 戻り値の型;
}
implementsとは?
Implements(インプリメンツ)は、クラスがInterfaceで宣言されたメソッドを実装することを示します。
クラスはInterfaceのメソッドをすべて実装しなければなりません。
<?php
class クラス名 implements インターフェース名
{
public function 関数名(): 戻り値の型
{
// 関数の実行内容
}
}
スポンサードサーチ
ControllerでInterfaceとimplementsを使った例
Interfaceの定義
HogeInterface
というInterfaceを定義します。
// app/Contracts/HogeInterface.php
namespace App\Contracts;
interface HogeInterface
{
public function getHoge(): string;
}
Interfaceは、サービスプロバイダで使用するために「app/Contracts」ディレクトリに配置します。
Interfaceの実装(implementsの使用)
Interfaceを実装するクラスを作成します。
// app/Services/HogeService.php
namespace App\Services;
use App\Contracts\HogeInterface;
class HogeService implements HogeInterface
{
public function getHoge(): string
{
return 'hoge';
}
}
「app/Services
」ディレクトリにHogeService
クラスを作成します。
AppServiceProviderでバインディング
AppServiceProvider(プロバイダ)でInterfaceと実装クラスをバインディングします。
// app/Providers/AppServiceProvider.php
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use App\Contracts\HogeInterface;
use App\Services\HogeService;
class AppServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
$this->app->bind(HogeInterface::class, HogeService::class);
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
//
}
}
app/Providers/AppServiceProvider.php
を編集します。
AppServiceProvider
とは?
AppServiceProvider
は、Laravelアプリケーションのコア部分を構成し、様々なサービス(依存関係)の登録と設定を行います。
「AppServiceProviderはapp/Providers」ディレクトリにあり、「Illuminate\Support\ServiceProvider」クラスを拡張しています。
- サービスコンテナへのバインディング:
- サービスプロバイダは、インターフェースとその具体的な実装をサービスコンテナにバインドするために使用されます。これにより、依存性注入が可能となり、柔軟な設計が可能となります。
- イベントのリスニング:
- サービスプロバイダは、イベントリスナーを登録するためにも使用されます。
- サービスの初期化:
- アプリケーション起動時に必要な初期化処理を行うために使用されます。
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
// サービスのバインディングを行う場所
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
// アプリケーションの初期化処理を行う場所
}
}
Controllerでinterfaceを使用
コントローラーでHogeInterface
を使用します。
// app/Http/Controllers/HogeController.php
namespace App\Http\Controllers;
use App\Contracts\HogeInterface;
class HogeController extends Controller
{
protected $hogeService;
public function __construct(HogeInterface $hogeService)
{
$this->hogeService = $hogeService;
}
public function show()
{
$result = $this->hogeService->getHoge();
return response()->json(['message' => $result]);
}
}
「app/Http/Controllers/HogeController.php」を作成します。
ルートの定義
ルートを定義して、コントローラーのメソッドを呼び出せるようにします。
// routes/web.php
use App\Http\Controllers\HogeController;
Route::get('/hoge', [HogeController::class, 'show']);
「/hoge」でHogeControllerのshowメソッドが実行されます。
結果
ブラウザで「https://laravel-app/hoge」にアクセスすると、HogeControllerのshowメソッドが呼び出され、HogeServiceのgetHogeメソッドの結果が表示されます。
{
"message": "hoge"
}
まとめ
Interface(HogeInterface
)を定義し、それを実装するサービス(HogeService
)を作成し、サービスプロバイダでバインディングしました。Controller
(HogeController
)でそのサービスを使用して、エンドポイントにアクセスしたときに結果を返すようにしました。
この設計により、サービスの実装を変更する場合でも、Interfaceを通じて簡単に置き換えることができ、コードの柔軟性と保守性が向上します。
スポンサードサーチ
ServiceでInterfaceとimplementsを使った例
Interfaceの定義
UserRepositoryInterface
という名前のInterfaceを作成します。
// app/Repositories/UserRepositoryInterface.php
namespace App\Repositories;
interface UserRepositoryInterface
{
public function find($id);
public function all();
}
Interfaceの実装(implementsの使用)
Interfaceを実装するUserRepository
クラスを作成します。
// app/Repositories/UserRepository.php
namespace App\Repositories;
use App\Models\User;
class UserRepository implements UserRepositoryInterface
{
public function find($id)
{
return User::find($id);
}
public function all()
{
return User::all();
}
}
AppServiceProviderでバインディング
AppServiceProvider(プロバイダ)でInterfaceと実装クラスをバインディングします。
// app/Providers/AppServiceProvider.php
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use App\Repositories\UserRepositoryInterface;
use App\Repositories\UserRepository;
class AppServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->bind(UserRepositoryInterface::class, UserRepository::class);
}
public function boot()
{
//
}
}
Serviceクラスでinterfaceを使用
Serviceクラスを作成して、リポジトリを利用します。
// app/Services/UserService.php
namespace App\Services;
use App\Repositories\UserRepositoryInterface;
class UserService
{
protected $userRepository;
public function __construct(UserRepositoryInterface $userRepository)
{
$this->userRepository = $userRepository;
}
public function getUserById($id)
{
return $this->userRepository->find($id);
}
public function getAllUsers()
{
return $this->userRepository->all();
}
}
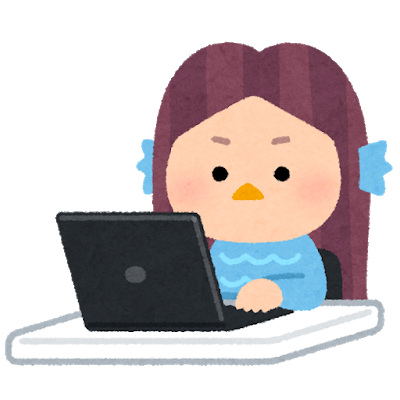
Interfaceのを使ったサービス関数のまとめです
- getUserById($id):UserRepositoryを使用して指定されたIDのユーザーを取得
- getAllUsers():UserRepositoryを使用して全ユーザーを取得
ControllerでServiceの使用
ControllerでServiceクラスを利用します。
// app/Http/Controllers/UserController.php
namespace App\Http\Controllers;
use App\Services\UserService;
class UserController extends Controller
{
protected $userService;
public function __construct(UserService $userService)
{
$this->userService = $userService;
}
public function show($id)
{
$user = $this->userService->getUserById($id);
return response()->json($user);
}
public function index()
{
$users = $this->userService->getAllUsers();
return response()->json($users);
}
}
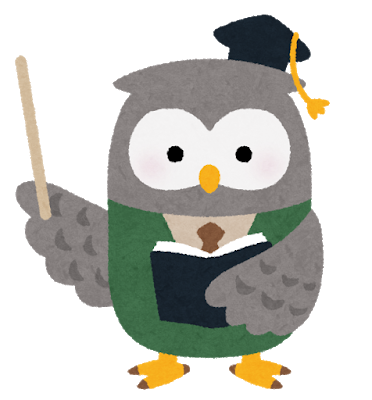
Serviceを使ったサービス関数のまとめです
- show($id):UserServiceを使って指定されたIDのユーザー情報を取得
- index():UserServiceを使って全ユーザー情報を取得
ルートの定義
ルートを定義して、コントローラーのメソッドを呼び出せるようにします。
// routes/web.php
use App\Http\Controllers\UserController;
// 全てのユーザー情報を取得するルート
Route::get('/users', [UserController::class, 'index']);
// 特定のユーザー情報を取得するルート
Route::get('/users/{id}', [UserController::class, 'show']);
結果
URIが/users/の場合
ブラウザで「https://laravel-app/users」にアクセスする、UserControllerのindexメソッドが実行され、
userServiceクラスのgetAllUsersメソッドが実行されます。
getAllUsersメソッドでは、UserRepositoryInterfaceのallメソッドを実行しています。
結果、JSONデータが返ってきます。
※データベースの構造による(省略)
URIが/users/{id}の場合
ブラウザで「https://laravel-app/users/{id}」にアクセスする、UserControllerのshowメソッドが実行され、
userServiceクラスのgetUserByIdメソッドが実行されます。
getUserByIdメソッドでは、UserRepositoryInterfaceのfindメソッドを実行しています。
結果、JSONデータが返ってきます。
※データベースの構造による(省略)
まとめ
- Interface(UserRepositoryInterface)を作成し、それを実装するクラス(UserRepository)を定義しました。
- ServiceプロバイダでInterfaceと実装クラスをバインディングし、Serviceクラス(UserService)でリポジトリを利用しました。
- Controller(UserController)でServiceクラスを使用しました。